Data modelling with Prisma
on this page
We need a database to store our Posts, and Users in our blog. In this tutorial, we will be using Prisma, and a simple sqlite file for the sake of simplicity. First we need to define our models or tables, and some basic requirements.
Prisma setup
- Install some of the basic dependencies such as typescript, ts-node(or tsx) etc. Then install prisma as a dev dependency.
npm install prisma --save-dev
- Go ahead and initialize prisma. This will create the schema.prisma file for you to add your schemas to, and also setup and sqlite connection.
npx prisma init --datasource-provider sqlite
User model
A user should have a name, unique id, and an email. They should also have a password that they will use to register, and login.
We can use the following schema for our User in the schema.prisma file.
model User {
id Int @id @default(autoincrement())
email String @unique
name String
password String
posts Post[]
}
One thing to notice is the posts attribute of type Post[]. This is basically setting up a one-to-many relation. One User can have many Posts. We will define the Post model next.
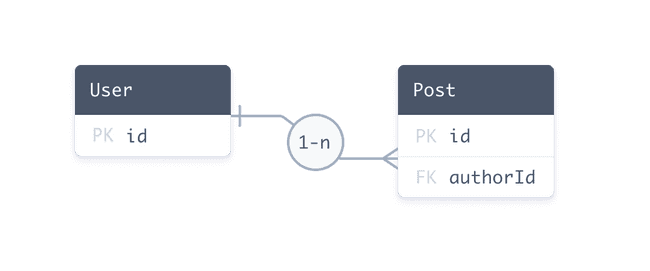
Post model
A post should have a unique id, title, content, date of creation, and an author. Add the following schema to schema.prisma.
model Post {
id Int @id @default(autoincrement())
title String
content String
creationDate DateTime @default(now())
author User @relation(fields: [authorId], references: [id])
authorId Int
}
The author field here represents the relationship between Post, and User. In terms of sql, authorId is the foreign key referencing the attribute User.id.
Migration
Now we will use prisma migrate
to populate the physical sqlite file with the tables that we have defined. Remember that we must rerun prisma migrate every time we make a change to the schemas.
npx prisma migrate dev --name init
Queries and mutations
Now we are ready to use the prisma client, and perform any types of queries, or mutations with our models. Heres an example of creating a user.
import { PrismaClient } from "@prisma/client";
const prisma = new PrismaClient();
prisma.user.create({ data : {
name : "John",
email : "John@doe.com",
password : "IamJohnDoe"
}}).then(user => {
console.log(user);
});